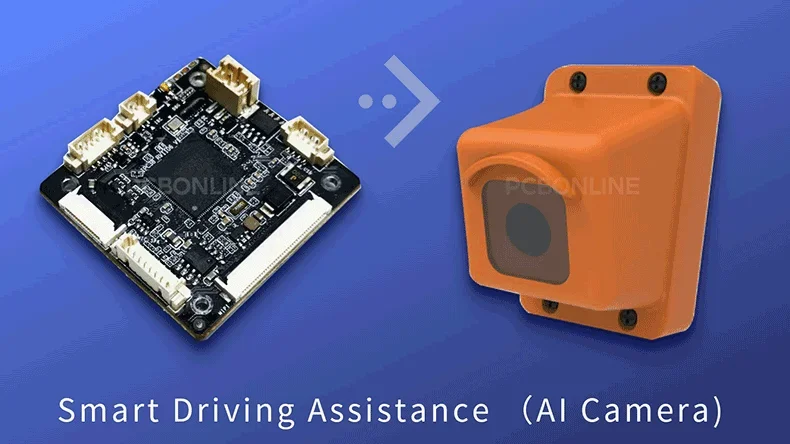
Bluetooth Low Energy (BLE) is transforming how we connect devices in the Internet of Things (IoT). Its low power consumption and short-range capabilities make it ideal for battery-operated devices like wearables and smart home gadgets. The ESP32 is a powerful microcontroller that integrates Wi-Fi and BLE, making it a popular choice for developers.
In this article, we'll walk you through BLE with ESP32 with an example — creating a BLE server that sends simulated temperature data using the ESP32.
In this article:
What is BLE with ESP32? Example Project: Set Up Your ESP32 BLE Temperature Sensor Applications of BLE with ESP32 One-stop IoT PCBA Manufacturer PCBONLINEWhat is BLE with ESP32?
Whether you're a hobbyist looking to experiment with BLE or a professional developing a complex system, the ESP32 provides a robust platform for BLE-based applications across various industries.
What is BLE?
Bluetooth Low Energy (BLE) is part of the Bluetooth 4.0 specification and is designed for low power consumption. It allows devices to communicate efficiently over short distances, typically 10 to 100 meters. Here are some key features of BLE:
- Low power consumption: Perfect for battery-operated devices that must last long.
- Quick connections: BLE devices can connect quickly, making them suitable for applications where speed is essential.
- Multiple device connections: BLE can handle multiple connections at once, allowing for a mesh of interconnected devices.
Why Choose the ESP32?
The ESP32 is a versatile microcontroller that combines Wi-Fi and BLE capabilities. The below features make the ESP32 an excellent choice for a wide range of IoT projects.
- Dual-core processing power
- Ample GPIO pins
- Integrated Wi-Fi and Bluetooth
- Rich library support
By using the ESP32's BLE capabilities, you can build a wide variety of applications that require real-time data transmission with low power consumption. The ESP32's versatility, combined with BLE functionality, makes it an excellent choice for IoT applications.
Example Project: Set Up Your ESP32 BLE Temperature Sensor
Below, we use a BLE temperature sensor with the ESP32 prototype project as an example to illustrate how to set up BLE with ESP32.
Requirement
- An ESP32 development board
- An Arduino IDE with ESP32 board support installed
- A BLE client app on your smartphone (like nRF Connect)
- A real temperature sensor, such as DHT11 or LM35
Schematic
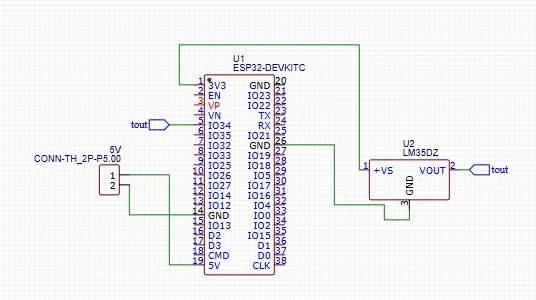
PCB Design
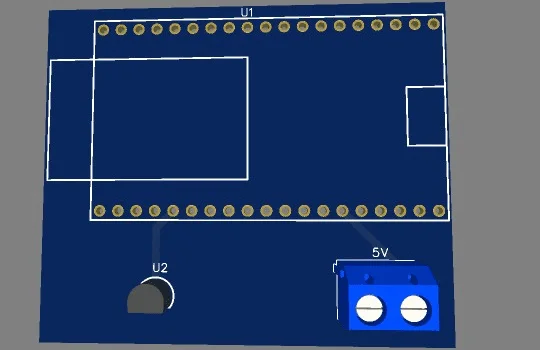
Step-by-step guide
1. Install the ESP32 Board in Arduino IDE
- Open the Arduino IDE and go to File > Preferences.
- In the "Additional Board Manager URLs," add:
https://dl.espressif.com/dl/package_esp32_index.json - Then, go to Tools > Board > Board Manager, search for ESP32, and install the package.
2. Connect your ESP32 Board
Connect your ESP32 board to your computer using a USB cable.
3. Write the BLE server code
Create a new sketch in the Arduino IDE and paste the following code:
#include <Arduino.h>
#include <BLEDevice.h>
#include <BLEUtils.h>
#include <BLEServer.h>
#include <BLE2902.h> // Explicitly include BLE2902
const int lm35Pin = 34; // Connect the LM35 to pin 34 of the ESP32
BLECharacteristic *pCharacteristic;
bool deviceConnected = false;
// UUIDs for the BLE Service and Characteristic
#define SERVICE_UUID "4fafc201-1fb5-459e-8fcc-c5c9c331914b"
#define CHARACTERISTIC_UUID "beb5483e-36e1-4688-b7f5-ea07361b26a8"
// Function to read temperature from the LM35 sensor
float readTemperature() {
int sensorValue = analogRead(lm35Pin);
float millivolts = (sensorValue / 4095.0) * 3300; // ESP32 ADC resolution is 12-bit
float temperatureC = millivolts / 10; // LM35 outputs 10 mV per degree Celsius
return temperatureC;
}
class MyServerCallbacks : public BLEServerCallbacks {
void onConnect(BLEServer* pServer) {
deviceConnected = true;
}
void onDisconnect(BLEServer* pServer) {
deviceConnected = false;
}
};
void setup() {
Serial.begin(115200);
// Initialize BLE
BLEDevice::init("ESP32_Temperature_Sensor");
BLEServer *pServer = BLEDevice::createServer();
pServer->setCallbacks(new MyServerCallbacks());
// Create the BLE Service
BLEService *pService = pServer->createService(SERVICE_UUID);
// Create the BLE Characteristic
pCharacteristic = pService->createCharacteristic(
CHARACTERISTIC_UUID,
BLECharacteristic::PROPERTY_READ |
BLECharacteristic::PROPERTY_NOTIFY
);
pCharacteristic->addDescriptor(new BLE2902());
// Start the service
pService->start();
// Start advertising
BLEAdvertising *pAdvertising = BLEDevice::getAdvertising();
pAdvertising->addServiceUUID(SERVICE_UUID);
pAdvertising->setScanResponse(true);
pAdvertising->setMinPreferred(0x06); // Set the advertising interval
BLEDevice::startAdvertising();
Serial.println("Waiting for a client to connect...");
}
void loop() {
if (deviceConnected) {
float temperature = readTemperature();
char tempString[8];
dtostrf(temperature, 4, 2, tempString); // Convert temperature to string with 2 decimal places
pCharacteristic->setValue(tempString); // Set temperature value as characteristic value
pCharacteristic->notify(); // Notify connected device
Serial.print("Temperature: ");
Serial.println(tempString);
}
delay(1000); // Update every second
}
4. Upload the Code
Select the correct board and port in the Arduino IDE and upload the code to your ESP32.
5. Testing the BLE server
Use a BLE client app: Download a BLE client app like nRF Connect from the Google Play Store on your smartphone.
Connect to your ESP32: Open the app and look for a device named "ESP32_Temperature_Sensor". Connect to it.
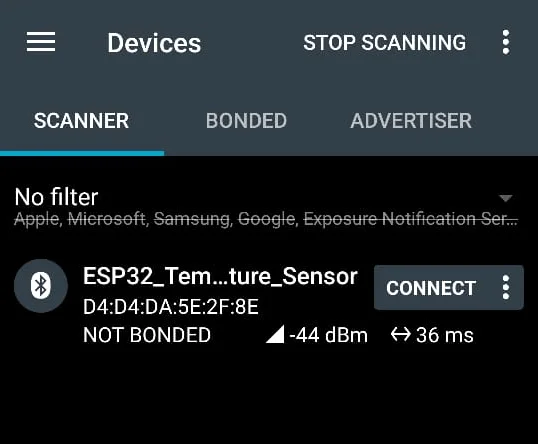
Subscribe to notifications: Once connected, subscribe to the notifications for the temperature characteristic. You should start receiving updates every 1 seconds.
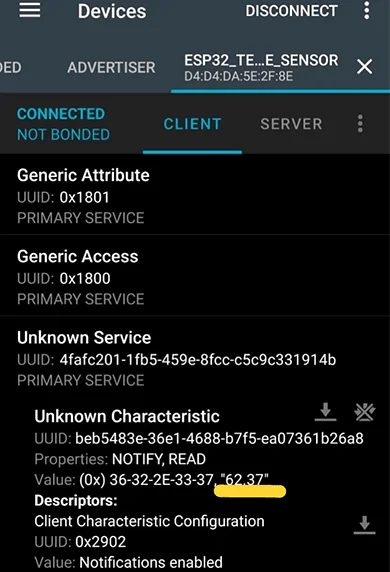
This project demonstrates how easy it is to collect environmental data, like temperature, and send it wirelessly to mobile devices, enabling real-time monitoring and alerts. With the ESP32 and LM35, developers can create scalable solutions that bridge the gap between sensors and mobile applications.
Applications of BLE with ESP32
The combination of BLE with ESP32 has opened up a myriad of possibilities for various applications. Below are some common use cases where BLE with ESP32 shines:
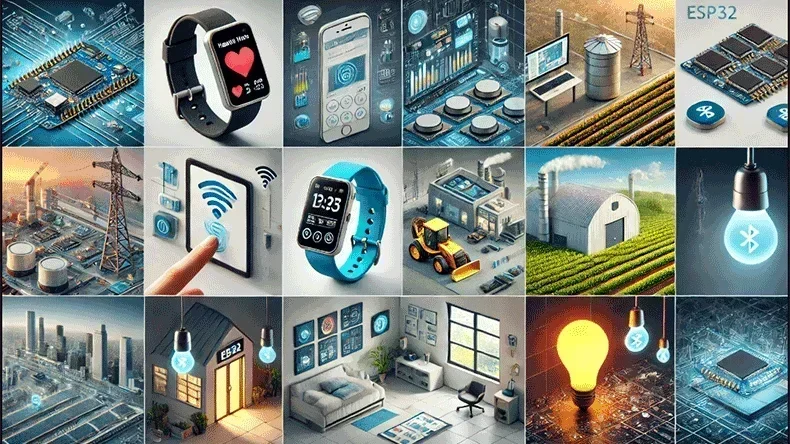
Health and fitness devices
One of the most popular use cases for BLE is in health and fitness devices. BLE sensors can be used to measure things like heart rate, body temperature, blood pressure, and more. The ESP32 can collect this data and send it to a smartphone or cloud server for further processing and analysis.
Example application: A wearable health monitor that measures heart rate and body temperature in real time and syncs the data to a mobile app using BLE.
Smart home automation
The ESP32, with its BLE functionality, can be used as the central hub in a smart home network. BLE allows for energy-efficient communication between devices like smart light bulbs, thermostats, door locks, and security cameras. Since BLE is a low-power protocol, it can be used in battery-powered devices that need to run for extended periods.
Example application: A BLE-enabled smart thermostat that communicates with a mobile app or a central control system to adjust the temperature in a home based on user preferences.
Industrial IoT (IIoT) and asset tracking
BLE is widely used in asset tracking and industrial monitoring. With ESP32, you can build systems that collect data from sensors in factories, warehouses, or supply chains and transmit this data to cloud platforms for analysis. BLE tags can be attached to assets, allowing for real-time location tracking and inventory management.
Example application: A BLE-based asset tracking system that monitors the location and status of equipment in a factory or warehouse, sending data to a central monitoring system powered by ESP32.
Smart agriculture
In smart agriculture, BLE with ESP32 can be used to create sensor networks for monitoring soil moisture, temperature, and other environmental factors. This data can be sent to a cloud server or a mobile app for farmers to make informed decisions about irrigation, fertilization, and crop management.
Example application: A BLE-enabled soil moisture sensor powered by an ESP32 that transmits soil moisture data to a cloud platform for analysis and alerts when irrigation is needed.
Proximity sensing and beacons
BLE beacons are small, low-power devices that can broadcast signals to nearby devices. ESP32, with its BLE capabilities, can act as a beacon to send out information about location, promotions, or even weather updates to nearby users' smartphones.
Example application: A retail store using BLE beacons (powered by ESP32) to send location-based offers or promotions to customers' smartphones as they walk through different aisles.
Home security systems
ESP32 can be integrated into security systems, using BLE to wirelessly connect sensors (motion detectors, door/window sensors, cameras) to a central hub. BLE offers the advantage of low power, so battery-operated security sensors can work for extended periods without needing frequent recharging.
Example application: A BLE-enabled door/window sensor that connects wirelessly to an ESP32-powered home security system, sending alerts to the user's smartphone if the door or window is opened.
Smart lighting systems
Smart lighting systems are another excellent application for BLE with ESP32. The ESP32 can be used to control and monitor the state of lights, including adjusting brightness, changing colors, and turning lights on or off. The BLE communication protocol ensures that these lights can be controlled by a mobile app with low power consumption.
Example application: A BLE-enabled smart bulb controlled by an ESP32 microcontroller, which allows users to adjust light settings remotely via a mobile app.
One-stop IoT PCBA Manufacturer PCBONLINE
If you want R&D and one-stop electronics manufacturing to turn your BLE with ESP32 project into real IoT devices, you can work with the turnkey IoT PCBA manufacturer PCBONLINE.
Founded in 1999, PCBONLINE has two large advanced PCB manufacturing bases, one PCB assembly factory, stable supply chains, and an R&D team.
PCBONLINE has one-stop electronics manufacturing capabilities for IoT devices, including R&D, prototyping/sampling, PCB fabrication, component sourcing, PCB assembly, PCBA value-added, and IoT device box-build assembly.
PCBONLINE has strategic cooperation with Espressif for sourcing the ESP32, ESP8266, and other BLE with IoT microcontrollers conveniently.
The professionals at PCBONLINE offer one-on-one engineering support and free design for manufacturing (DFM) to ensure smooth manufacturing and successful results of your BLE with IoT project.
High-quality IoT PCBA manufacturing certified with ISO9001:2015, ISO14001:2015, IATF16949:2016, RoHS, REACH, UL, and IPC-A-610 Class 2/3.
Offers free prototyping/sampling, PCBA functional testing, and R&D for BLE with ESP32 massive production.
No matter what applications your IoT project will serve, such as industrial, automotive, consumer electronics, etc, and no matter what quantity you want, PCBONLINE can work for you from prototype to finished device box-builds. To get a quote for your BLE with ESP32 project, contact info@pcbonline.com.
Conclusion
In this article, we demonstrated how to create a simple BLE server using the ESP32 that sends simulated temperature data to a connected client. The ESP32's BLE capabilities open up a wide range of possibilities for IoT applications, from health monitoring and smart homes to industrial IoT and asset tracking. BLE's low power consumption, combined with the versatility of the ESP32 microcontroller, makes it an ideal solution for building efficient, long-lasting wireless devices. PCBONLINE is an IoT PCBA manufacturer providing R&D and electronics manufacturing for BLE with ESP32 projects under one roof. If you want to turn your BLE with ESP32 project or idea into real devices, chat with PCBONLINE from the online chat window.
PCB assembly at PCBONLINE.pdf